Enzyme and Mocha Test For React Sample
TDD for React
1.Enzyme
## Shallow Rendering
1 2 3 4
| import { shallow } from 'enzyme';
const wrapper = shallow(<MyComponent />); // ...
|
## Full Rendering
1 2 3 4
| import { mount } from 'enzyme';
const wrapper = mount(<MyComponent />); // ...
|
## Static Rendering
1 2 3 4
| import { render } from 'enzyme';
const wrapper = render(<MyComponent />); // ...
|
2.Chai
* Chai has several interfaces that allow the developer to choose the most comfortable.
## Should
1 2 3 4 5 6
| chai.should();
foo.should.be.a('string'); foo.should.equal('bar'); foo.should.have.length(3); tea.should.have.property('flavors').with.length(3);
|
## Expect
1 2 3 4 5 6
| var expect = chai.expect;
expect(foo).to.be.a('string'); expect(foo).to.equal('bar'); expect(foo).to.have.length(3); expect(tea).to.have.property('flavors').with.length(3);
|
## Assert
1 2 3 4 5 6 7
| var assert = chai.assert;
assert.typeOf(foo, 'string'); assert.equal(foo, 'bar'); assert.lengthOf(foo, 3) assert.property(tea, 'flavors'); assert.lengthOf(tea.flavors, 3);
|
—————————————–source———————————————–
1. packaget.json
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| { "name": "enzyme-example-mocha", "version": "0.1.0", "description": "Example project with React + Enzyme + Mocha", "main": "build/index.js", "scripts": { "test": "mocha test/.setup.js test/**/*-test.js" }, "repository": { "type": "git", "url": "https://github.com/lelandrichardson/enzyme-example-mocha.git" }, "author": "Leland Richardson <leland.richardson@airbnb.com>", "license": "MIT", "bugs": { "url": "https://github.com/lelandrichardson/enzyme-example-mocha/issues" }, "homepage": "https://github.com/lelandrichardson/enzyme-example-mocha", "devDependencies": { "babel": "^6.3.26", "babel-preset-airbnb": "^1.0.1", "babel-register": "^6.4.3", "chai": "^3.5.0", "enzyme": "^2.0.0", "jsdom": "^8.0.1", "mocha": "^2.4.5", "react-addons-test-utils": "^0.14.7" }, "dependencies": { "react": "^0.14.7", "react-dom": "^0.14.7" } }
|
2.Foo.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import React, { PropTypes } from 'react';
const propTypes = {};
const defaultProps = {};
class Foo extends React.Component { constructor(props) { super(props); }
render() { return ( <div className="foo" /> ); } }
Foo.propTypes = propTypes; Foo.defaultProps = defaultProps;
export default Foo;
|
3. Foo-test.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| import React from 'react'; import { expect } from 'chai'; import { shallow, mount, render } from 'enzyme'; import Foo from '../src/Foo';
describe("A suite", function() { it("contains spec with an expectation", function() { expect(shallow(<Foo />).contains(<div className="foo" />)).to.equal(true); });
it("contains spec with an expectation", function() { expect(shallow(<Foo />).is('.foo')).to.equal(true); });
it("contains spec with an expectation", function() { expect(mount(<Foo />).find('.foo').length).to.equal(1); }); });
|
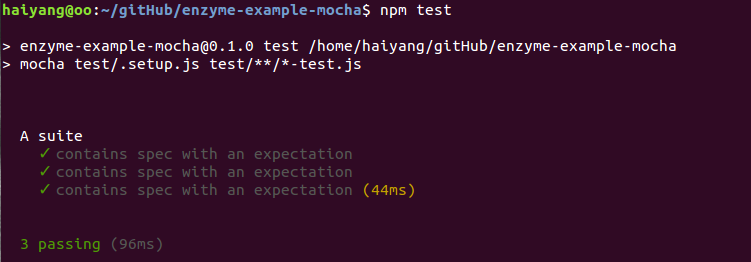