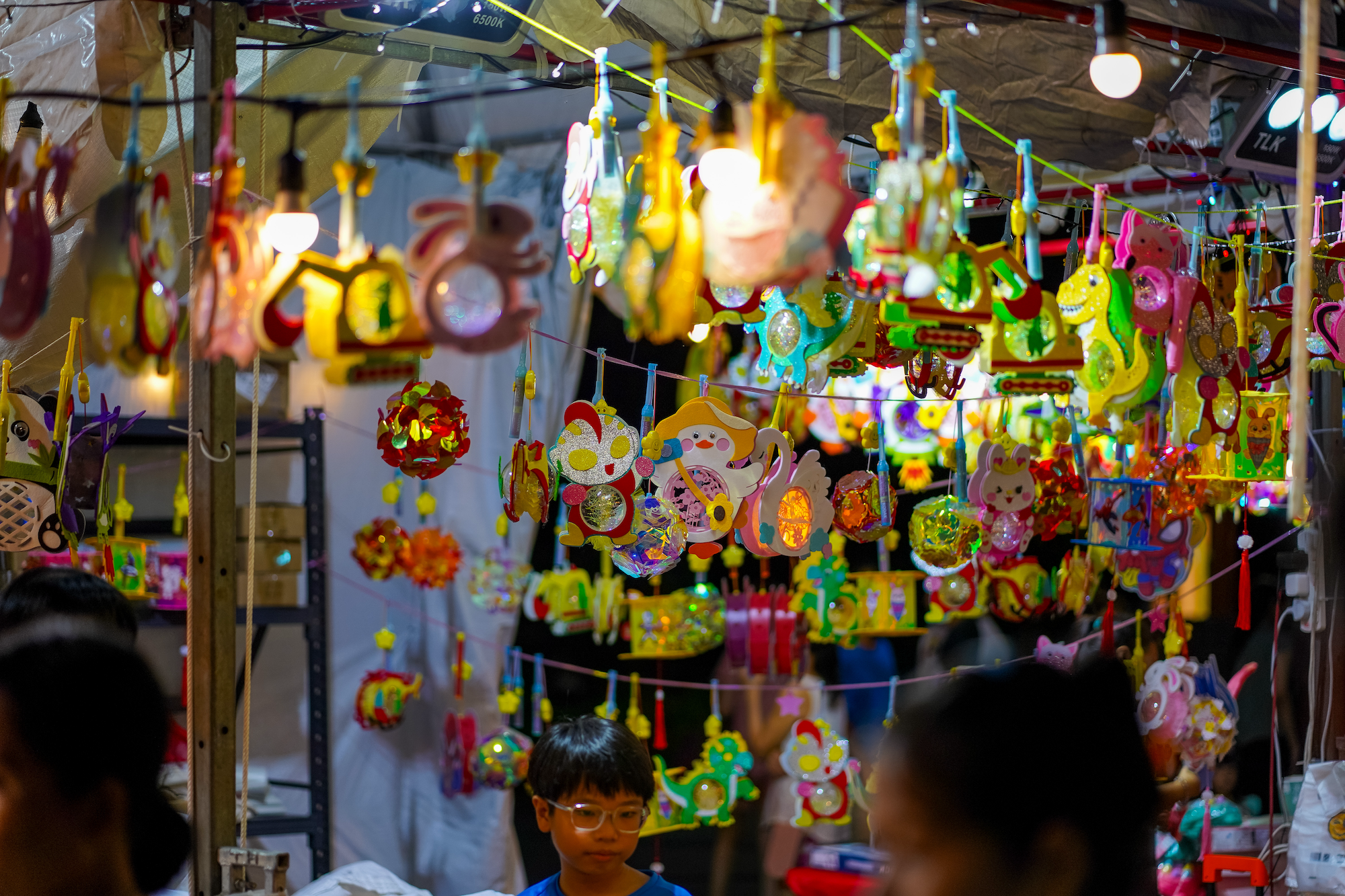
Here are some JavaScript tricks that can be handy:
1. Destructuring Assignment:
1 2
| const person = { name: 'John', age: 30 }; const { name, age } = person;
|
2. Spread Operator:
1 2
| const arr1 = [1, 2, 3]; const arr2 = [...arr1, 4, 5, 6];
|
3. Ternary Operator:
1 2
| const age = 18; const status = (age >= 18) ? 'Adult' : 'Minor';
|
4. Template Literals:
1 2
| const name = 'John'; const greeting = `Hello, ${name}!`;
|
5. Arrow Functions:
1
| const double = (num) => num * 2;
|
6. Object Shorthand:
1 2 3
| const name = 'John'; const age = 30; const person = { name, age };
|
7. Default Parameters:
1 2 3
| function greet(name = 'User') { return `Hello, ${name}!`; }
|
8. Truthy and Falsy Values:
1 2 3 4 5 6 7 8 9 10
| const truthyExample = 'Hello'; const falsyExample = 0;
if (truthyExample) { console.log('Truthy'); }
if (!falsyExample) { console.log('Falsy'); }
|
9. Array.prototype.includes():
1 2
| const numbers = [1, 2, 3, 4, 5]; const includesThree = numbers.includes(3);
|
10. Array.prototype.find():
1 2 3 4 5 6
| const users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' }, { id: 3, name: 'Bob' } ]; const user = users.find(user => user.id === 2);
|
11. Array Destructuring:
1 2
| const colors = ['red', 'green', 'blue']; const [firstColor, secondColor] = colors;
|
12. Rest Parameter:
1 2 3
| function sum(...numbers) { return numbers.reduce((acc, num) => acc + num, 0); }
|
13. Object Destructuring with Default Values:
1
| const { name = 'John', age = 30 } = person;
|
14. Array Spread Operator for Function Arguments:
1 2 3 4 5 6
| function addNumbers(a, b, c) { return a + b + c; }
const numbers = [1, 2, 3]; const sum = addNumbers(...numbers);
|
15. Short-circuit Evaluation for Conditional Logic:
1 2
| const isLoggedIn = true; isLoggedIn && console.log('User is logged in');
|
16. String.prototype.startsWith() and endsWith():
1 2 3
| const str = 'Hello, world'; const startsWithHello = str.startsWith('Hello'); const endsWithWorld = str.endsWith('world');
|
17. Array.prototype.map():
1 2
| const numbers = [1, 2, 3, 4]; const squaredNumbers = numbers.map(num => num ** 2);
|
18. Array.prototype.filter():
1 2
| const numbers = [1, 2, 3, 4]; const evenNumbers = numbers.filter(num => num % 2 === 0);
|
19. Array.prototype.reduce():
1 2
| const numbers = [1, 2, 3, 4]; const sum = numbers.reduce((acc, num) => acc + num, 0);
|
20. Truthy and Falsy Coalescing Operator (??):
1 2
| const user = null; const name = user ?? 'Guest';
|
21. Optional Chaining (?.):
1 2 3 4 5 6 7 8
| const user = { address: { street: '123 Main St', city: 'New York' } };
const city = user?.address?.city;
|
22. Async/Await for Asynchronous Operations:
1 2 3 4 5
| async function getData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; }
|